
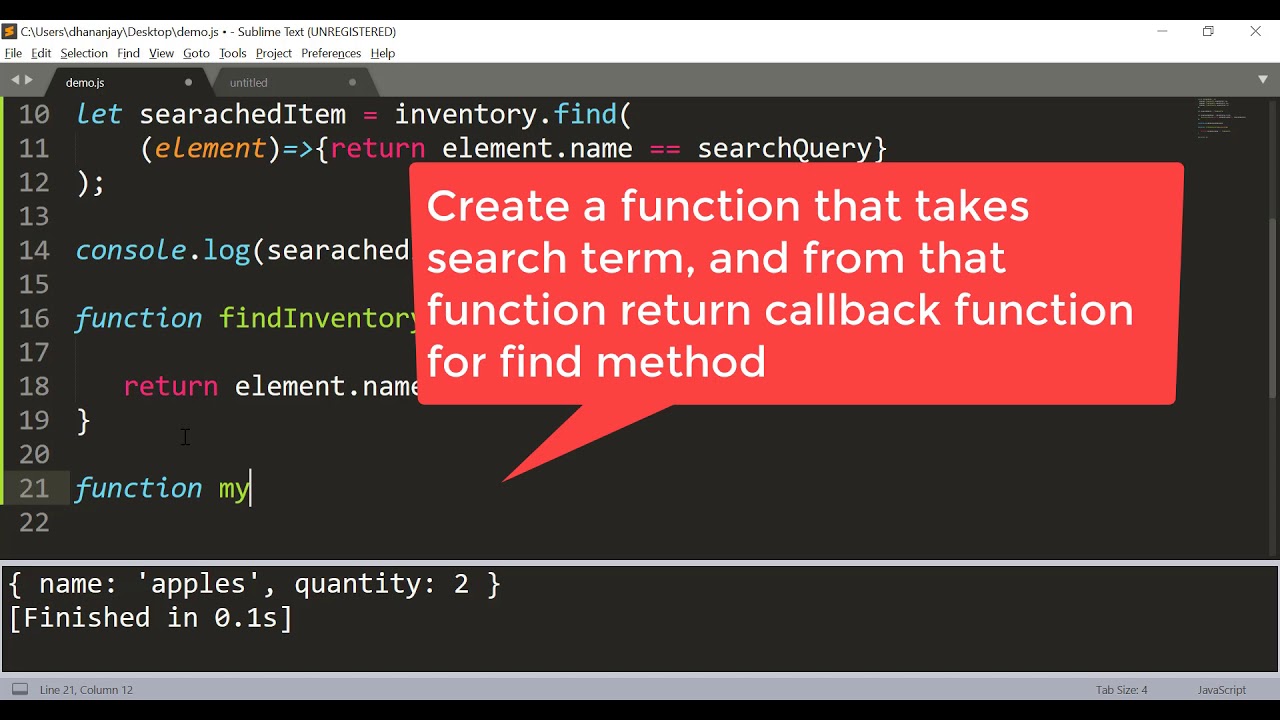
Some methods return items, while others may return an index or a Boolean.Array in JavaScript || Full-Stack Web-Development Course #24 1. Some search for explicit items, while others use a predicate to match. JavaScript contains many different methods for searching Arrays. Read the documentation for each method to discover all of these features.Įxample: const numbers = numbers.filter((item, index, array) => ) Summary Some of the predicates also include extra information like the index and the array. const numbers = const isEven = number => number % 2 = 0 const isLessThanThanZero = number => number true numbers.some(isLessThanThanZero) //=> false Extra Credit Takes a predicate and returns true if at least one items matches. const numbers = const isEven = number => number % 2 = 0 const isGreaterThanZero = number => number > 0 numbers.every(isEven) //=> false numbers.every(isGreaterThanZero) //=> true.
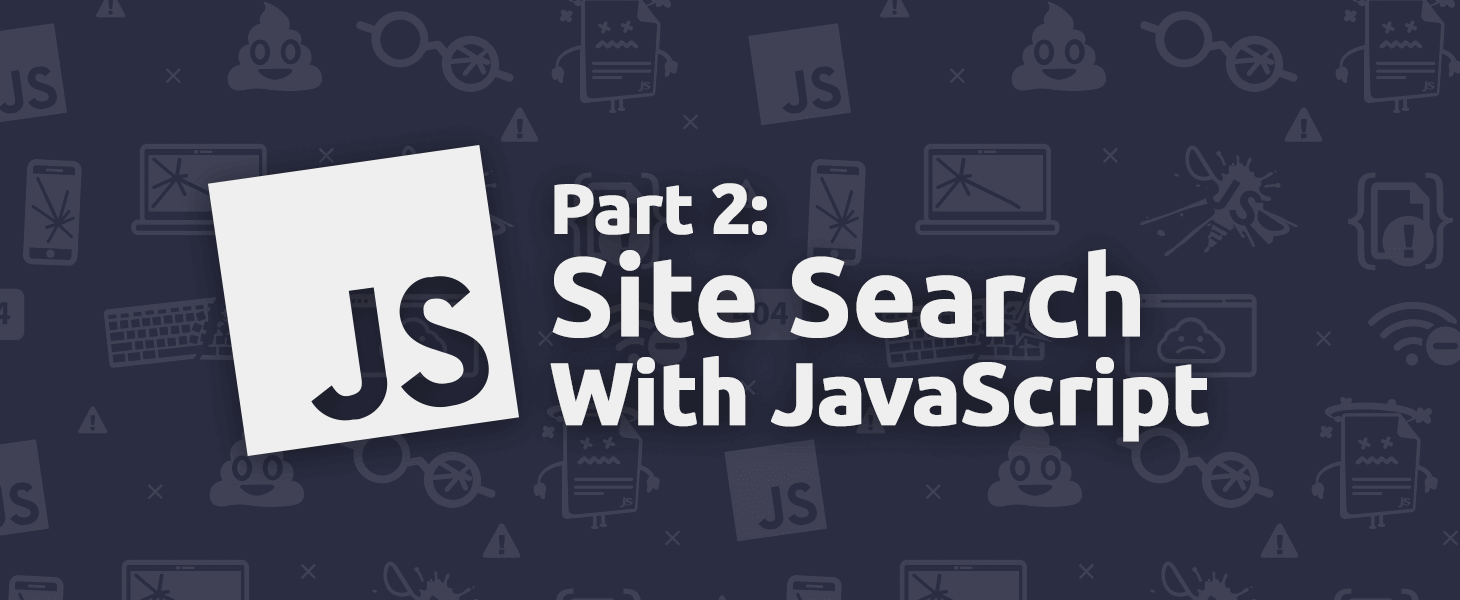
Takes a predicate and returns true if all items match. const numbers = numbers.includes(2) //=> true numbers.includes(9) //=> false. Takes an item and returns true if at least one item matches. Sometimes you only need to know whether or not items match.includes Same as indexOf, but searches in reverse. const numbers = numbers.indexOf(2) //=> 1 numbers.indexOf(9) //=> -1. Takes an item (and optional start) and returns the Index or -1. const numbers = const isEven = number => number % 2 = 0 const isLessThanZero = number => number 1 numbers.findIndex(isLessThanZero) //=> -1. Takes a predicate and returns the Index or -1. const numbers = const isEven = number => number % 2 = 0 const isLessThanZero = number => number 2 numbers.find(isLessThanZero) //=> undefined Find The Index of Matching Item. Takes a predicate and returns the first matching item or undefined. everyĪlso searches for multiple items, see implementation below. const numbers = const isEven = number => number % 2 = 0 const isLessThanZero = number => number numbers.filter(isLessThanZero) //=>.

Takes a predicate and returns an Array of all matching items. const isEven = number => number % 2 = 0 Searching for Multiple Items. The isEven function is an example of a predicate that takes a number and returns true if the number is even. A predicate is just a fancy word for a function that takes an item and returns a Boolean whether the item passes some condition.
